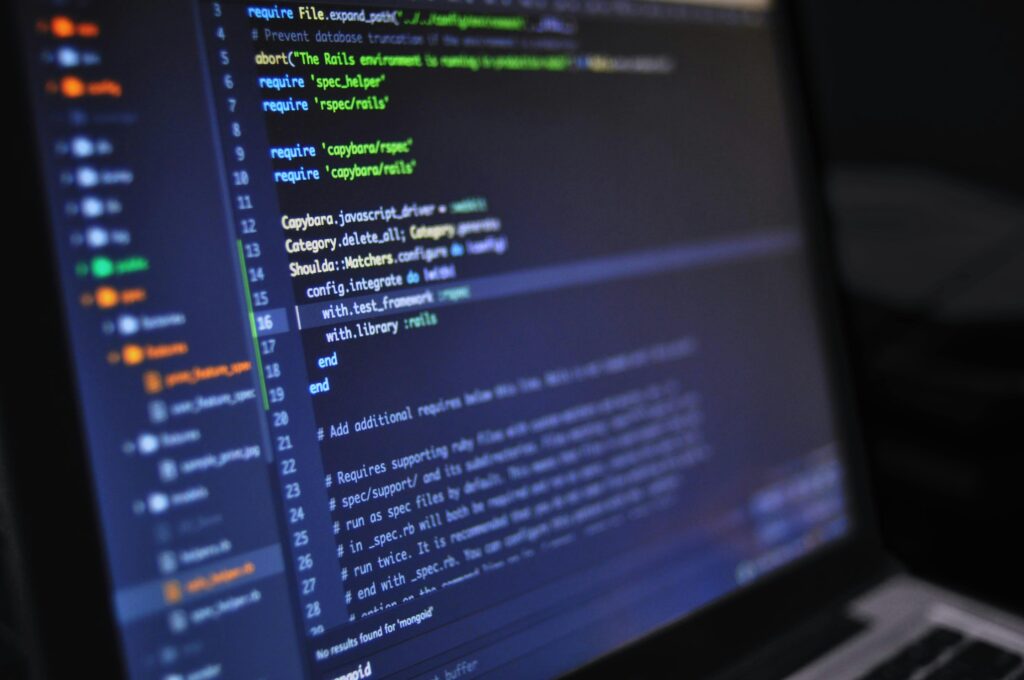
In computer science and algorithm design, categorized by their application and usage frequency. It highlights key concepts like Arrays, which are fundamental data structures, and advanced techniques such as Dynamic Programming, used for solving optimization problems. Topics like Sorting, Hash Tables, and Graphs cover essential methods for organizing and searching data efficiently. Techniques like Greedy Algorithms and Divide and Conquer represent strategies for solving problems step-by-step or by breaking them into smaller subproblems. Specialized methods like Backtracking, Sliding Window, and Monotonic Stacks are used for specific problem patterns.
The list also includes data structures like Binary Trees, Tries, and Segment Trees, which are used for hierarchical or range-based data queries. Algorithmic paradigms such as Recursion, Bit Manipulation, and Heap (Priority Queue) reflect different ways to approach computation. Other advanced topics, including Probability and Statistics, Game Theory, and Concurrency, extend into multidisciplinary applications. Finally, rare and niche areas like Eulerian Circuit, Biconnected Components, and Reservoir Sampling address specific challenges in graph theory and data sampling. Overall, the list is a comprehensive guide to the diverse tools and concepts used in problem-solving across domains.
Topics
- Arrays
- String
- Hash Table
- Dynamic Programming
- Math
- Sorting
- Greedy
- Depth-First Search
- Database
- Binary Search
- Matrix
- Tree
- Breadth-First Search
- Bit Manipulation
- Two Pointers
- Heap (Priority Queue)
- Prefix Sum
- Binary Tree
- Simulation
- Stack
- Counting
- Graph
- Sliding Window
- Design
- Backtracking
- Enumeration
- Union Find
- Linked List
- Ordered Set
- Number Theory
- Monotonic Stack
- Trie
- Segment Tree
- Bitmask
- Divide and Conquer
- Queue
- Recursion
- Combinatorics
- Binary Search Tree
- Hash Function
- Binary Indexed Tree
- Geometry
- Memoization
- String Matching
- Topological Sort
- Shortest Path
- Rolling Hash
- Game Theory
- Interactive
- Data Stream
- Monotonic Queue
- Brainteaser
- Randomized
- Merge Sort
- Doubly-Linked List
- Counting Sort
- Iterator
- Concurrency
- Probability and Statistics
- Quickselect
- Suffix Array
- Bucket Sort
- Minimum Spanning Tree
- Shell
- Line Sweep
- Reservoir Sampling
- Strongly Connected Component
- Eulerian Circuit
- Radix Sort
- Rejection Sampling
- Biconnected Component